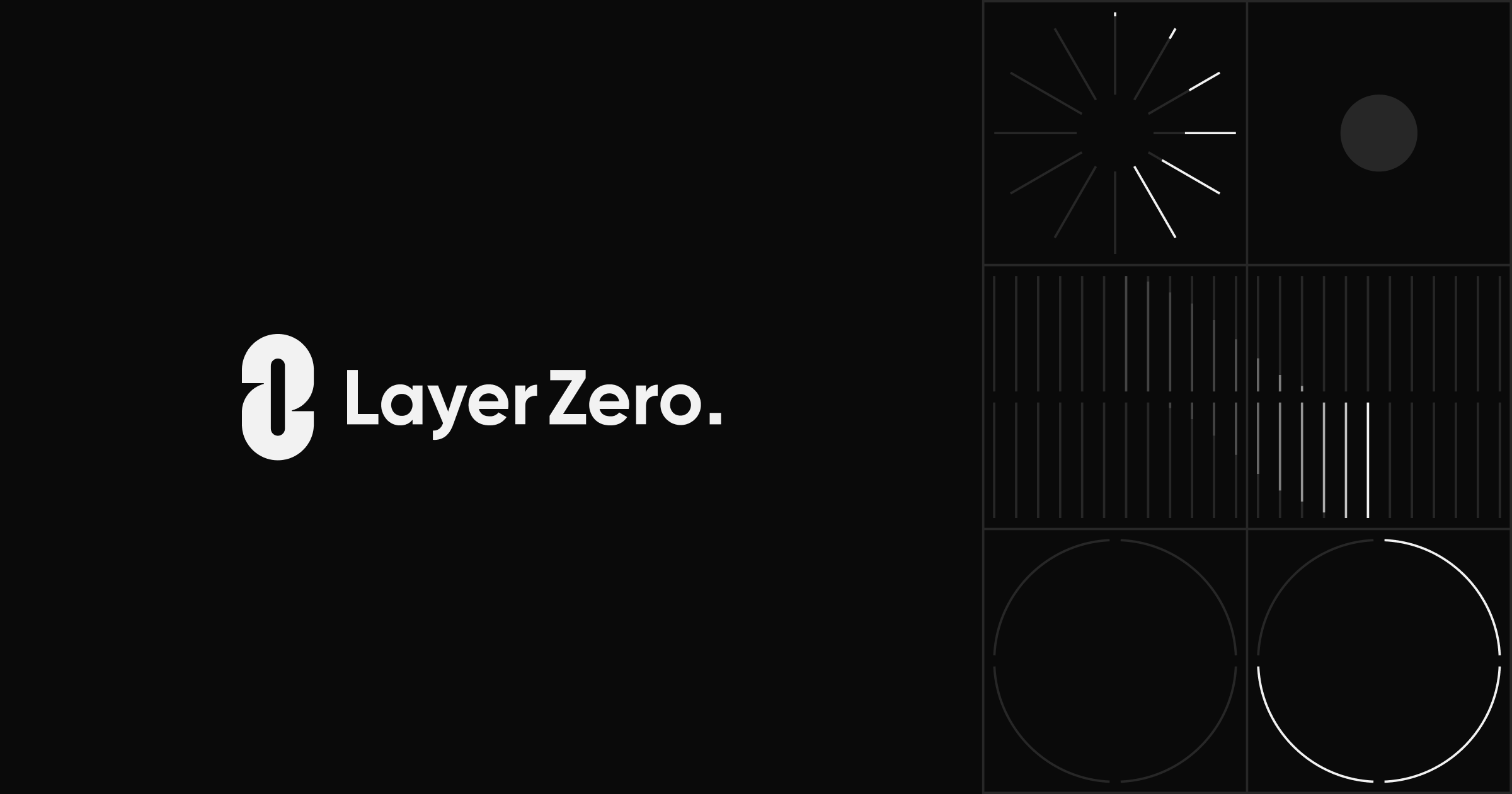
LayerZero: Revolutionizing Cross-Chain Interoperability
Young Kim / September 3, 2024
LayerZero is a revolutionary omnichain interoperability protocol that's reshaping the landscape of blockchain communication. This post delves deep into the technical intricacies of LayerZero, exploring its architecture, key components, and practical applications.
Understanding LayerZero's Architecture
At its core, LayerZero is designed to facilitate trustless cross-chain messaging between different blockchain networks. The protocol's architecture consists of several key components:
- Ultra Light Nodes (ULNs): These are lightweight, on-chain components that verify cross-chain messages.
- Oracles: Responsible for relaying block headers from the source chain to the destination chain.
- Relayers: Transmit proofs and payload data between chains.
contract LayerZeroEndpoint is ILayerZeroEndpoint {
mapping(uint16 => mapping(uint16 => bytes)) public ulns;
mapping(uint16 => address) public oracles;
mapping(uint16 => address) public relayers;
function send(
uint16 _dstChainId,
bytes calldata _destination,
bytes calldata _payload,
address payable _refundAddress,
address _zroPaymentAddress,
bytes calldata _adapterParams
) external payable override {
// Implementation details
}
// Other functions...
}
The Genius of Ultra Light Nodes
Ultra Light Nodes are a groundbreaking innovation in LayerZero's design. Unlike traditional light clients that require storing and validating block headers, ULNs leverage a combination of on-chain logic and off-chain proofs to achieve secure cross-chain communication with minimal on-chain footprint.
contract UltraLightNode {
function verifyProof(
uint16 _srcChainId,
bytes memory _srcAddress,
uint64 _nonce,
bytes memory _payload,
bytes memory _proof
) public view returns (bool) {
// Verification logic
}
}
Oracle and Relayer Dynamics
LayerZero's oracle and relayer system forms the backbone of its cross-chain messaging mechanism. Oracles provide block headers, while relayers submit proofs and payloads. This dual-prover model enhances security by requiring potential attackers to compromise both systems simultaneously.
interface ILayerZeroOracle {
function getBlockHeaderData(uint16 _chainId, uint64 _blockNumber) external view returns (bytes memory);
}
interface ILayerZeroRelayer {
function relayProof(
uint16 _srcChainId,
bytes memory _srcAddress,
uint64 _nonce,
bytes memory _payload,
bytes memory _proof
) external;
}
Implementing Cross-Chain Applications with LayerZero
Developing omnichain applications with LayerZero involves implementing the
ILayerZeroReceiver
interface and utilizing the LayerZeroEndpoint
for sending
messages.
contract CrossChainApplication is ILayerZeroReceiver {
ILayerZeroEndpoint public endpoint;
constructor(address _endpoint) {
endpoint = ILayerZeroEndpoint(_endpoint);
}
function sendCrossChainMessage(uint16 _dstChainId, bytes memory _payload) external payable {
endpoint.send{value: msg.value}(
_dstChainId,
abi.encodePacked(address(this)),
_payload,
payable(msg.sender),
address(0),
""
);
}
function lzReceive(
uint16 _srcChainId,
bytes memory _srcAddress,
uint64 _nonce,
bytes memory _payload
) external override {
require(msg.sender == address(endpoint), "Invalid endpoint caller");
// Process the received cross-chain message
}
}
Advanced Concepts: Adapters and Composability
LayerZero's adapter system allows for customizable message passing behavior, enabling developers to implement complex cross-chain logic:
interface ILayerZeroAdapter {
function send(
uint16 _dstChainId,
bytes memory _destination,
bytes memory _payload,
address payable _refundAddress,
address _zroPaymentAddress,
bytes memory _adapterParams
) external payable;
}
contract ComposableAdapter is ILayerZeroAdapter {
ILayerZeroAdapter[] public adapters;
function addAdapter(ILayerZeroAdapter _adapter) external {
adapters.push(_adapter);
}
function send(
uint16 _dstChainId,
bytes memory _destination,
bytes memory _payload,
address payable _refundAddress,
address _zroPaymentAddress,
bytes memory _adapterParams
) external payable override {
for (uint i = 0; i < adapters.length; i++) {
adapters[i].send{value: msg.value / adapters.length}(
_dstChainId,
_destination,
_payload,
_refundAddress,
_zroPaymentAddress,
_adapterParams
);
}
}
}
Security Considerations and Best Practices
When developing with LayerZero, it's crucial to implement proper security measures:
- Message Ordering: Ensure your application can handle out-of-order message delivery.
- Nonce Validation: Always validate the nonce to prevent replay attacks.
- Gas Management: Implement robust gas estimation and management for cross-chain transactions.
contract SecureLayerZeroApp is ILayerZeroReceiver {
mapping(uint16 => uint64) public lastNonce;
function lzReceive(
uint16 _srcChainId,
bytes memory _srcAddress,
uint64 _nonce,
bytes memory _payload
) external override {
require(_nonce > lastNonce[_srcChainId], "Invalid nonce");
lastNonce[_srcChainId] = _nonce;
// Process message
}
}
Conclusion
LayerZero represents a paradigm shift in blockchain interoperability. By providing a secure, efficient, and flexible protocol for cross-chain communication, it opens up new possibilities for decentralized applications and blockchain ecosystems. As the technology continues to evolve, we can expect to see increasingly sophisticated omnichain applications leveraging LayerZero's capabilities.